Frames iOS SDK
Our Frames iOS SDK allows you to accept online payments from all major credit cards and offers multiple levels of customization to suit your requirements.
Information
Our Frames iOS SDK is available on GitHub under the MIT license. For the SDK's API information, refer to the Frames iOS SDK API reference.
We stay up-to-date with the latest Payment Card Industry Data Security Standards (PCI DSS). Read our PCI compliance documentation to learn how we can help you to be compliant.
Based on these standards, we advise you to use one of our UI-based solutions of the Frames SDKs to remain at the lowest level of compliance (SAQ-A).
If you build your own UI, or use the Frames SDKs in another way, you may end up needing to adhere to a higher level of compliance.
- Integrate our Frames iOS SDK into your application's source code.
- Display the payment forms we provide to collect your customer's payment information and exchange this for a secure token. This is the tokenization step.
- Use the secure token to make a payment request.
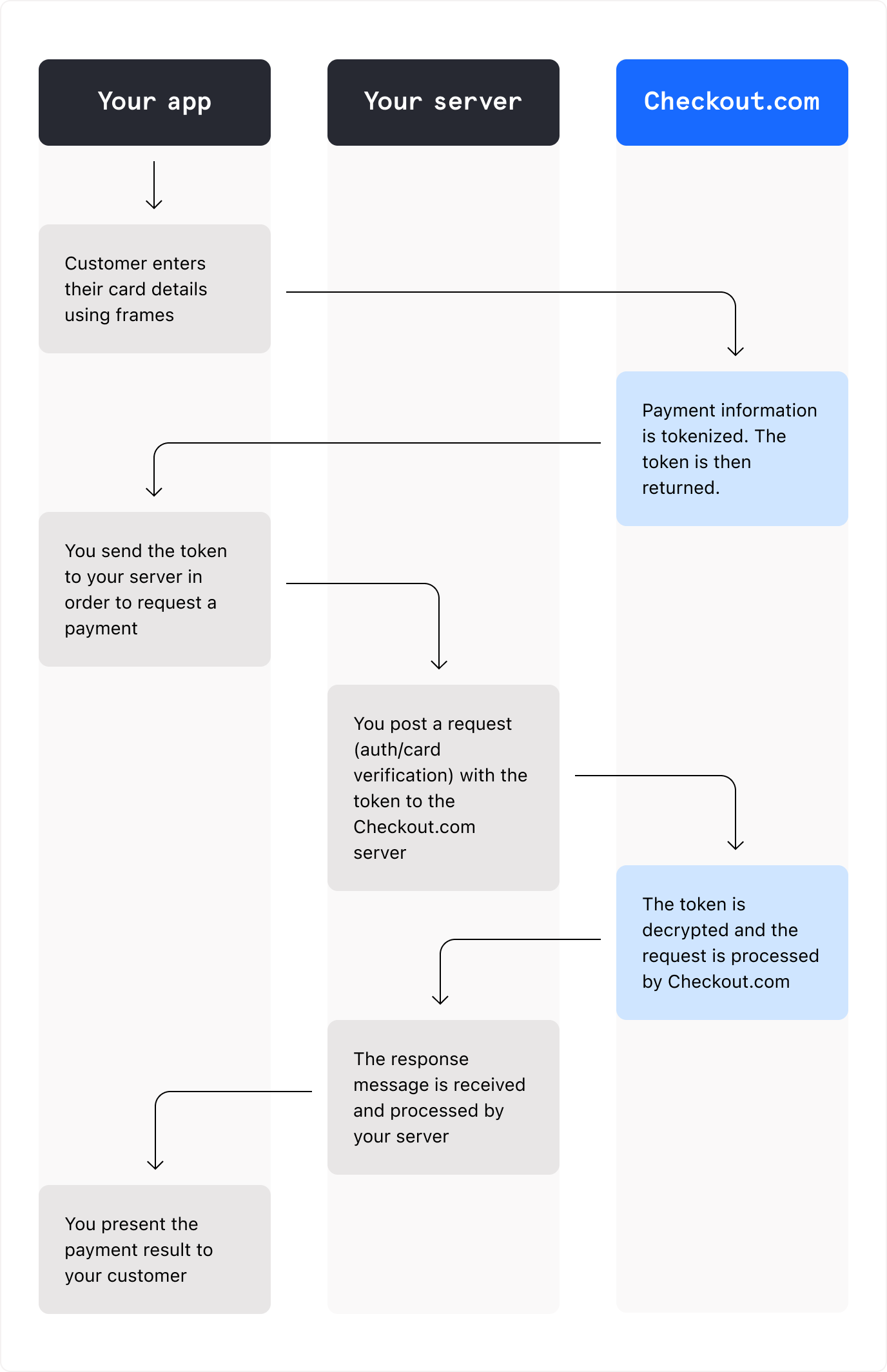
Before you follow this guide to integrate our Frames iOS SDK, make sure you have the following installed on your desktop:
- Swift 5.3 or later
- Xcode 12.4 or later, with iOS version 12 or later running in the simulator
We recommend using Swift Package Manager to add our SDK due to its ease of use, but we also support integration via the CocoaPods dependency manager.
Add our GitHub repository's URL (https://github.com/checkout/frames-ios
) as a package dependency in your app.
For detailed steps, follow the guide on Apple's documentation.
In a script in your project, add the following code:
1// Import the SDK2import Frames34// Defines customer information to for use in a new BillingForm object5let country = Country(iso3166Alpha2: "GB")67let address = Address(8addressLine1: "123 High St.",9addressLine2: "Flat 456",10city: "London",11zip: "SW1A 1AA",12country: country)1314let phone = Phone(number: "+44 1234567890",15country: country)1617// Creates a new BillingForm and populates it with the customer data defined above18let billingFormData = BillingForm(19name: "John Smith",20address: address,21phone: phone)2223/* Configures the payment environment. Providing billingFormData is optional,24but doing so can simplify your customer's checkout experience and improves the25chances of successful tokenization */2627let configuration = PaymentFormConfiguration(28apiKey: "<Your Public Key>",29environment: .sandbox,30supportedSchemes: [.visa, .maestro, .mastercard],31billingFormData: billingFormData)3233// Configures tokenization success and failure states34let completion: ((Result<TokenDetails, TokenRequestError>) -> Void) = { result in35switch result {36case .failure(let failure):37if failure == .userCancelled {38/* Depending on needs, User Cancelled can be handled as an individual39failure to complete, an error, or simply a callback that control is returned */40print("User has cancelled")41} else {42print("Failed, received error", failure.localizedDescription)43}44case .success(let tokenDetails):45print("Success, received token", tokenDetails.token)46}47}4849// Displays the forms to the user to collect payment information50let framesViewController = PaymentFormFactory.buildViewController(51configuration: configuration,52style: PaymentStyle(53paymentFormStyle: DefaultPaymentFormStyle(),54billingFormStyle: DefaultBillingFormStyle()),55completionHandler: completion56)57navigationController.pushViewController(framesViewController, animated: true)
If you want to change the styling of the forms displayed to the customer, you can do so using the paymentFormStyle
and billingFormStyle
objects from the example in the previous step.
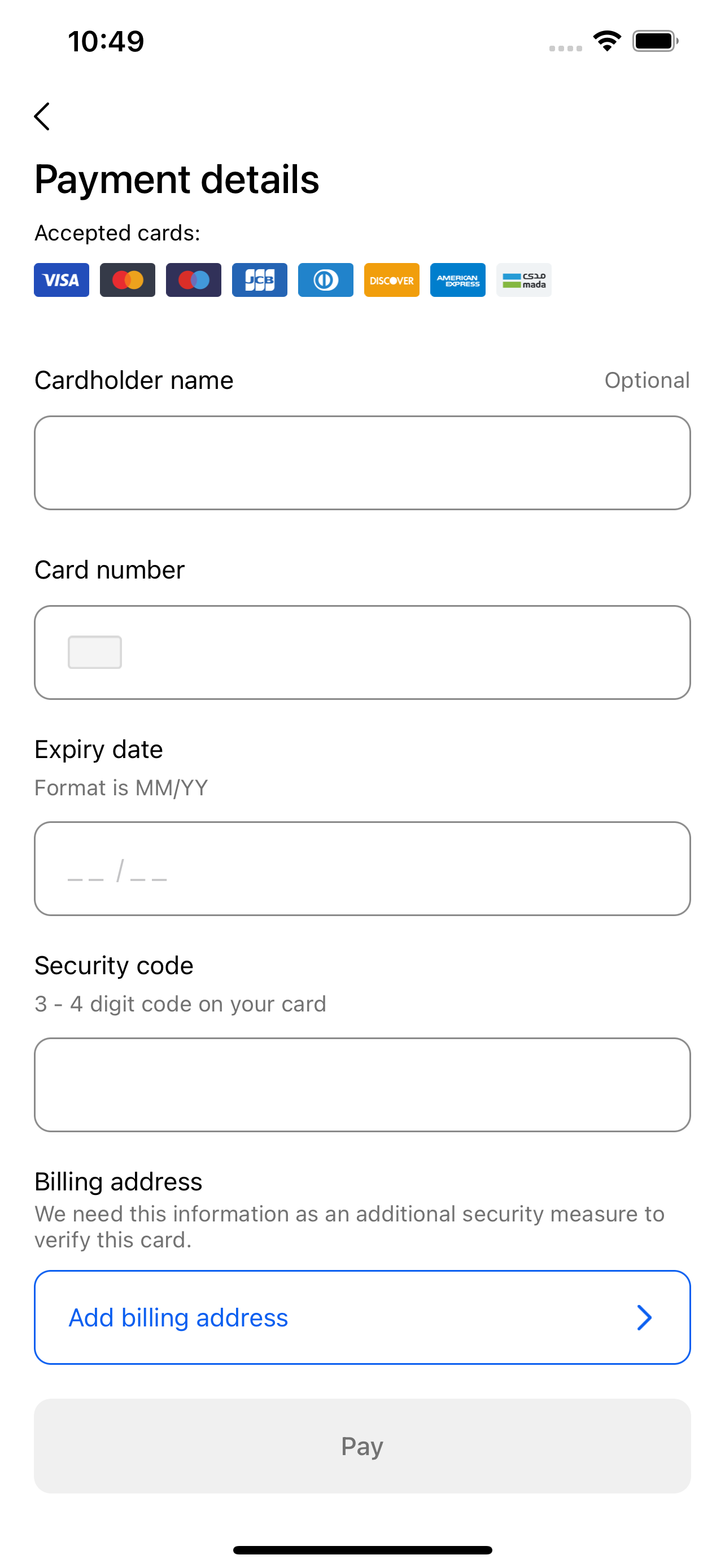
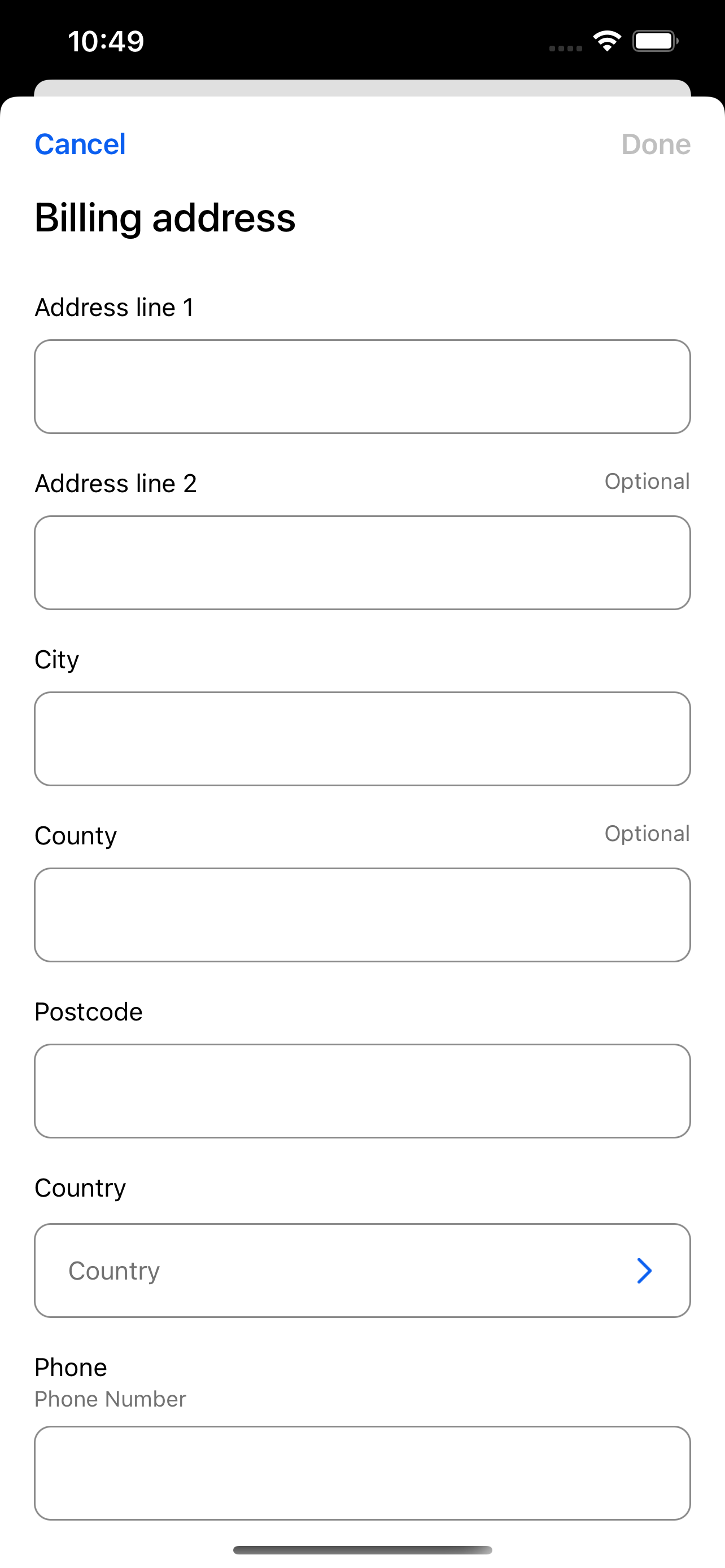
You can choose between three different options for customization, depending on how much control you need over the forms' UI design. In order of increasing complexity, these are:
- default form styling, which allows you to modify the fonts and font colors used in the default forms
- themed form styling, which allows you to apply a custom theme to the preset components
- custom form styling, which allows you to build and define your own components
When you send a 3D secure charge request from your server, you will get back a 3D Secure URL. This is available from _links.redirect.href
within the JSON response. You can then pass the 3D Secure URL to a ThreedsWebViewController
in order to handle the verification.
The redirection URLs (success_url
and failure_url
) are set in the Dashboard, but they can be overwritten in the charge request sent from your server. It is important to provide the correct URLs to ensure a successful payment flow.
1let threeDSWebViewController = ThreedsWebViewController(2successUrl: "https://example.com/success",3failUrl: "https://example.com/failure")4threeDSWebViewController.url = "https://example.com/3ds"5threeDSWebViewController.delegate = self
1extension ViewController: ThreedsWebViewControllerDelegate {23func threeDSWebViewControllerAuthenticationDidSucceed(_ threeDSWebViewController: ThreedsWebViewController, token: String?) {4// Handle successful 3DS.5}67func threeDSWebViewControllerAuthenticationDidFail(_ threeDSWebViewController: ThreedsWebViewController) {8// Handle failed 3DS.9}1011}
Our Frames iOS SDK also supports handling PKPayment
token data from Apple Pay.
1func handle(payment: PKPayment) {2// Create a CheckoutAPIClient instance with your public key.34let checkoutAPIClient = CheckoutAPIClient(5publicKey: "<Your Public Key>",6environment: .sandbox)78// Get the data containing the encrypted payment information.9let paymentData = payment.token.paymentData1011// Request an Apple Pay token.12checkoutAPIClient.createApplePayToken(paymentData: paymentData) { result in13switch result {14case .success(let response):15print(response)16case .failure(let error):17print(error.localizedDescription)18}19}20}
Read more about offering Apple Pay in your app.
Now that you have the card token, you can request a payment.