Frames Android SDK
Our Frames Android SDK allows you to accept online payments from all major credit cards and offers multiple levels of customization to suit your requirements.
Information
Our Frames Android SDK is available on GitHub under the MIT license. For the SDK's API information, refer to the Frames Android SDK API reference.
We stay up-to-date with the latest Payment Card Industry Data Security Standards (PCI DSS). Read our PCI compliance documentation to learn how we can help you to be compliant.
Based on these standards, we advise you to use one of our UI-based solutions of the Frames SDKs to remain at the lowest level of compliance (SAQ-A).
If you build your own UI, or use the Frames SDKs in another way, you may end up needing to adhere to a higher level of compliance.
- Integrate our Frames Android SDK into your application's source code.
- Display the payment forms we provide to collect your customer's payment information and exchange this for a secure token. This is the tokenization step.
- Use the secure token to make a payment request.
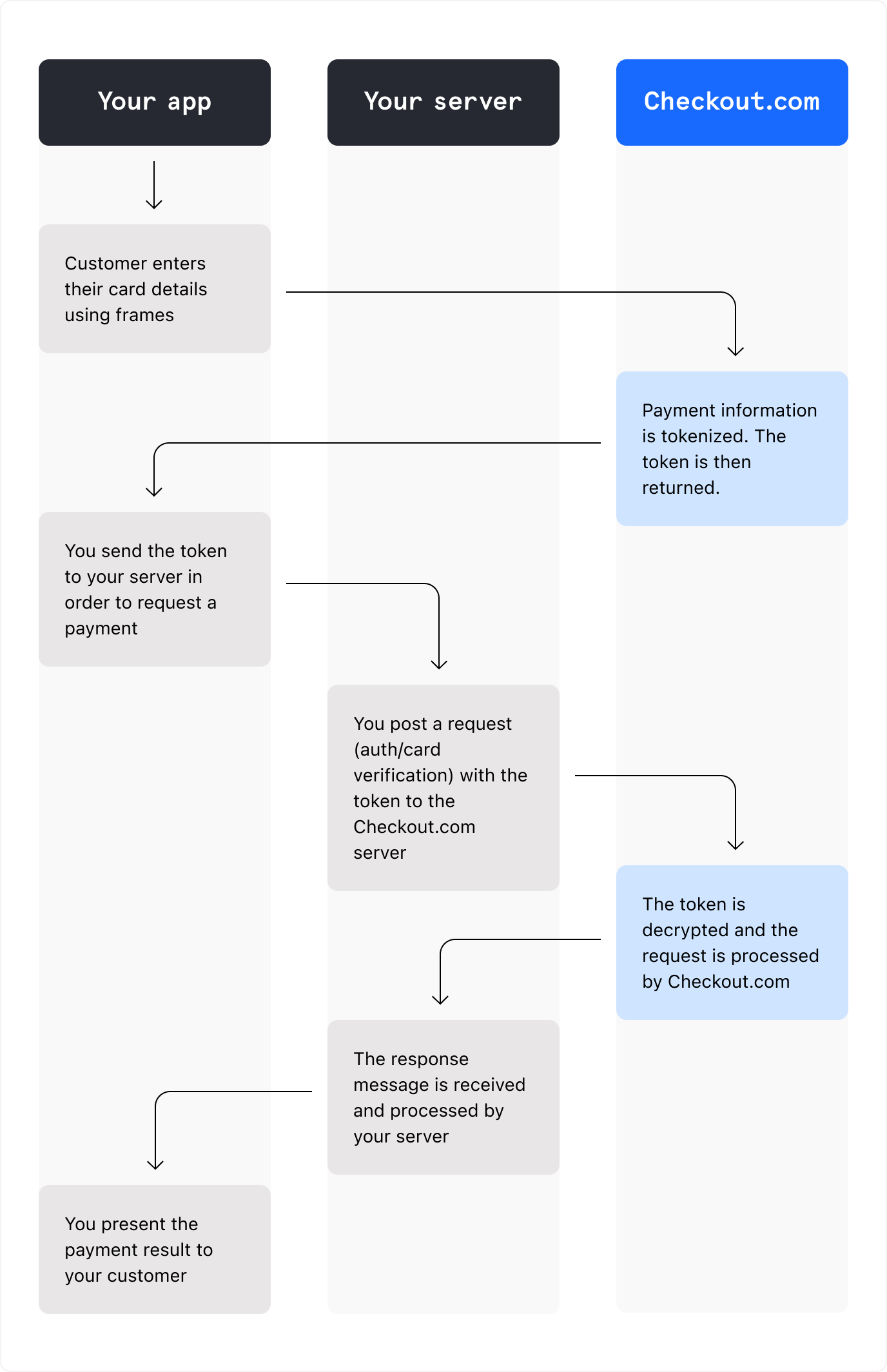
To integrate with our Frames Android SDK, you must be using Android API level 21 or later.
Information
You can find more about the installation and available versions on jitpack.io/frames-android.
Add JitPack repository to the project level build.gradle
file:
1// project gradle file2allprojects {3repositories {4maven { url 'https://jitpack.io' }5}6}
Add Frames SDK dependency to the module gradle file:
1// module gradle file2dependencies {3implementation 'com.github.checkout:frames-android:<latest_version>'4}
1val paymentFlowHandler = object : PaymentFlowHandler {2override fun onSubmit() {3// form submit initiated; you can choose to display a loader here4}56override fun onSuccess(tokenDetails: TokenDetails) {7// your token is here8}910override fun onFailure(errorMessage: String) {11// token request error12}1314override fun onBackPressed() {15// the user decided to leave the payment page16}17}
1val paymentFormConfig = PaymentFormConfig(2publicKey = PUBLIC_KEY, // set your public key3context = context, // set context4environment = Environment.SANDBOX, // set the environment5paymentFlowHandler = paymentFlowHandler, // set the callback6style = PaymentFormStyle(), // set the style7supportedCardSchemeList = emptyList() // set supported card schemes, by default uses all schemes8)
1val paymentFormMediator = PaymentFormMediator(paymentFormConfig)
1paymentFormMediator.PaymentForm() // Compose function
If you want to change the styling of the forms displayed to the customer, you can do so using the PaymentDetailsStyle
, BillingAddressDetailsStyle
and CountryPickerStyle
.
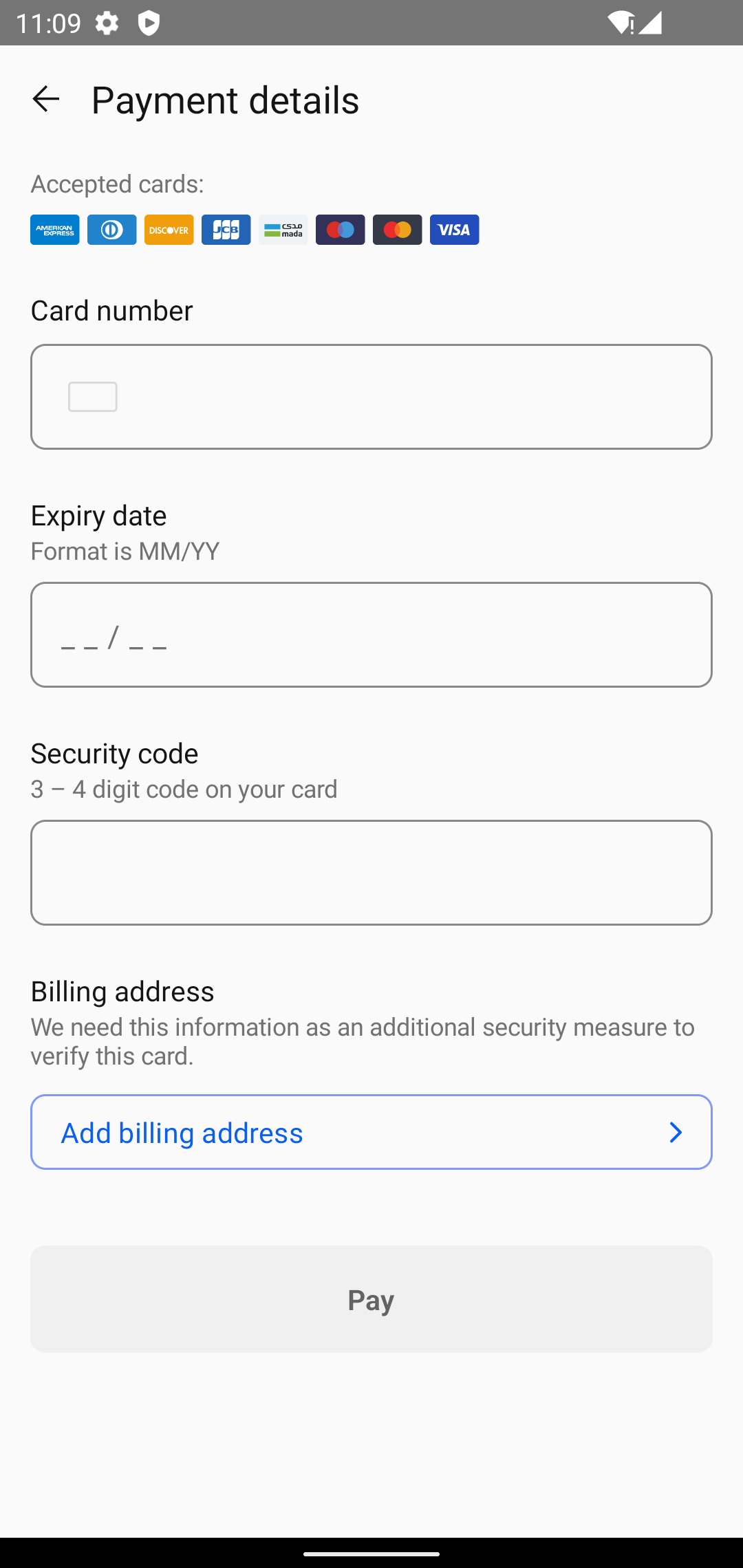
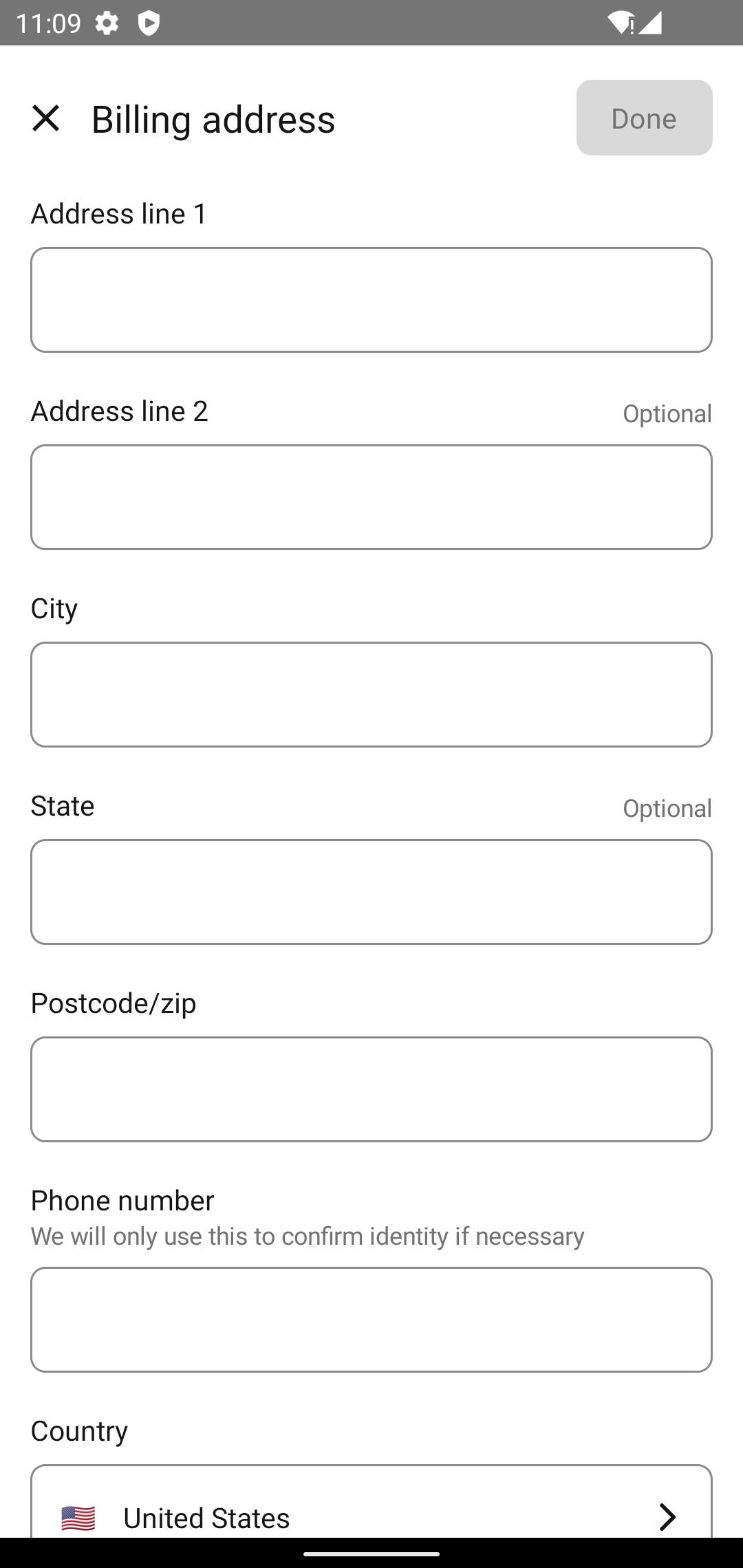
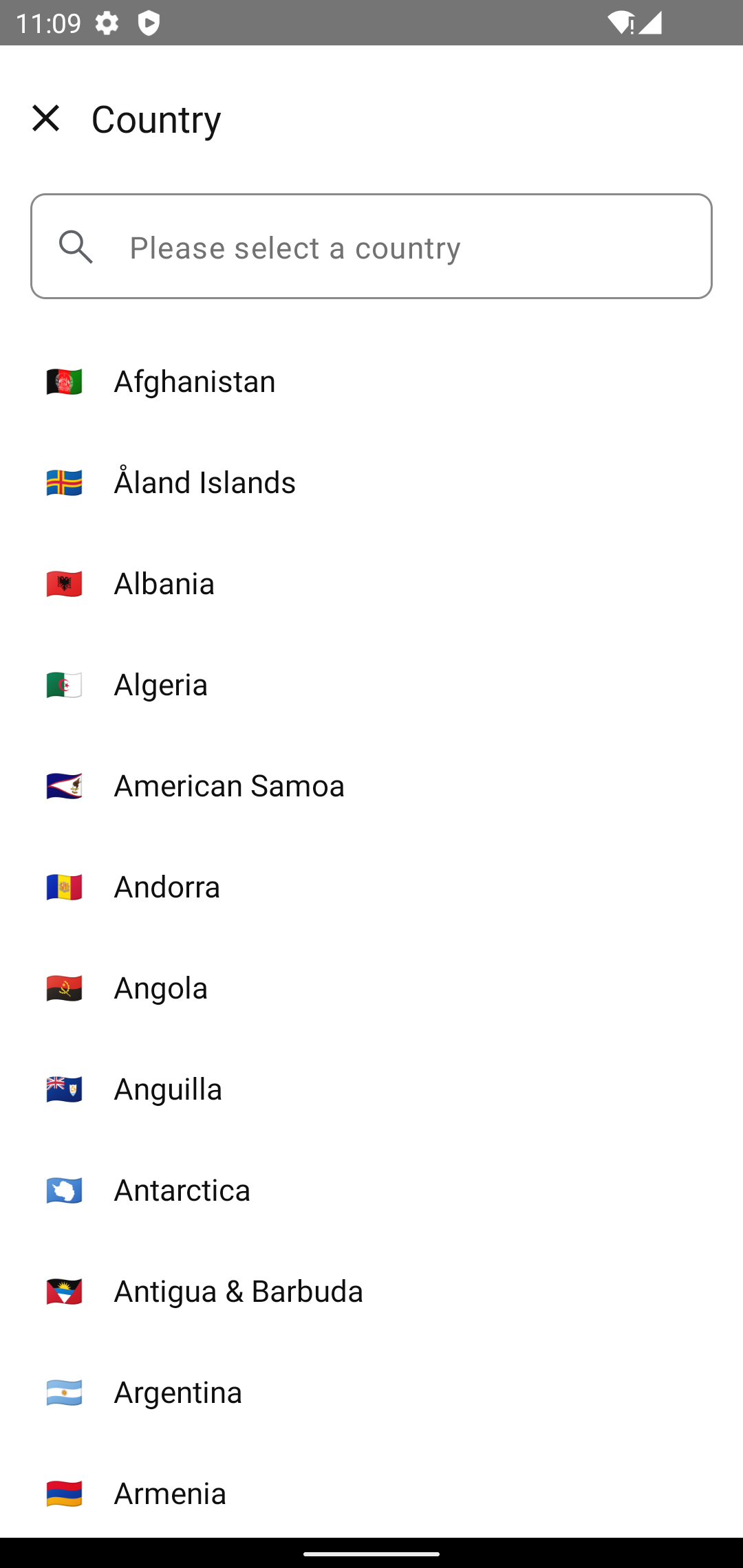
You can choose between three different options for customization, depending on how much control you need over the forms' UI design. In order of increasing complexity, these are:
- default form styling, which allows you to modify the fonts and font colors used in the default forms
- themed form styling, which allows you to apply a custom theme to the preset components
- custom form styling, which allows you to build and define your own components
When you send a 3D Secure charge request from your server, you will receive a 3D Secure URL in the response's _links.redirect.href
field. You can pass this URL to a PaymentFormMediator
in order to handle verification.
The redirection URLs, success_url
and failure_url
, are set in the Dashboard, but they can be overwritten in the charge request sent from your server. It's important that you provide the correct URLs to ensure a successful payment flow.
You can find an example of handling 3DS in the DemoActivity.java
file from the Frames Android SDK repository.
1val threeDSResultHandler: ThreeDSResultHandler = { threeDSResult: ThreeDSResult ->2when (threeDSResult) {3is ThreeDSResult.Success -> {4/* Handle success result */5threeDSResult.token6}7is ThreeDSResult.Failure -> {8/* Handle failure result */9}10is ThreeDSResult.Error -> {11/* Handle error result */12threeDSResult.error13}14}15}
1val request = ThreeDSRequest(2container = findViewById(android.R.id.content), // Provide a ViewGroup container for 3DS WebView3challengeUrl = redirectUrl, // Provide a 3D Secure URL4successUrl = "http://example.com/success",5failureUrl = "http://example.com/failure",6resultHandler = threeDSResultHandler7)8paymentFormMediator.handleThreeDS(request)
Handle a Google Pay token payload and retrieve a token, that you can use to create a charge from your back end.
1val checkoutApiClient = CheckoutApiServiceFactory.create(2publicKey = PUBLIC_KEY, // set your public key3environment = Environment.SANDBOX, // set context4context = context // set the environment5)
1val request = GooglePayTokenRequest(2tokenJsonPayload = tokenJsonPayload,3onSuccess = { tokenDetails ->4/* Handle success result */5tokenDetails.token6},7onFailure = { errorMessage ->8/* Handle failure result */9}10)11checkoutApiClient.createToken(request)
Now that you have the card token, you can request a payment.